Convert Milliseconds to Date: Understanding the Essential Data Transformation
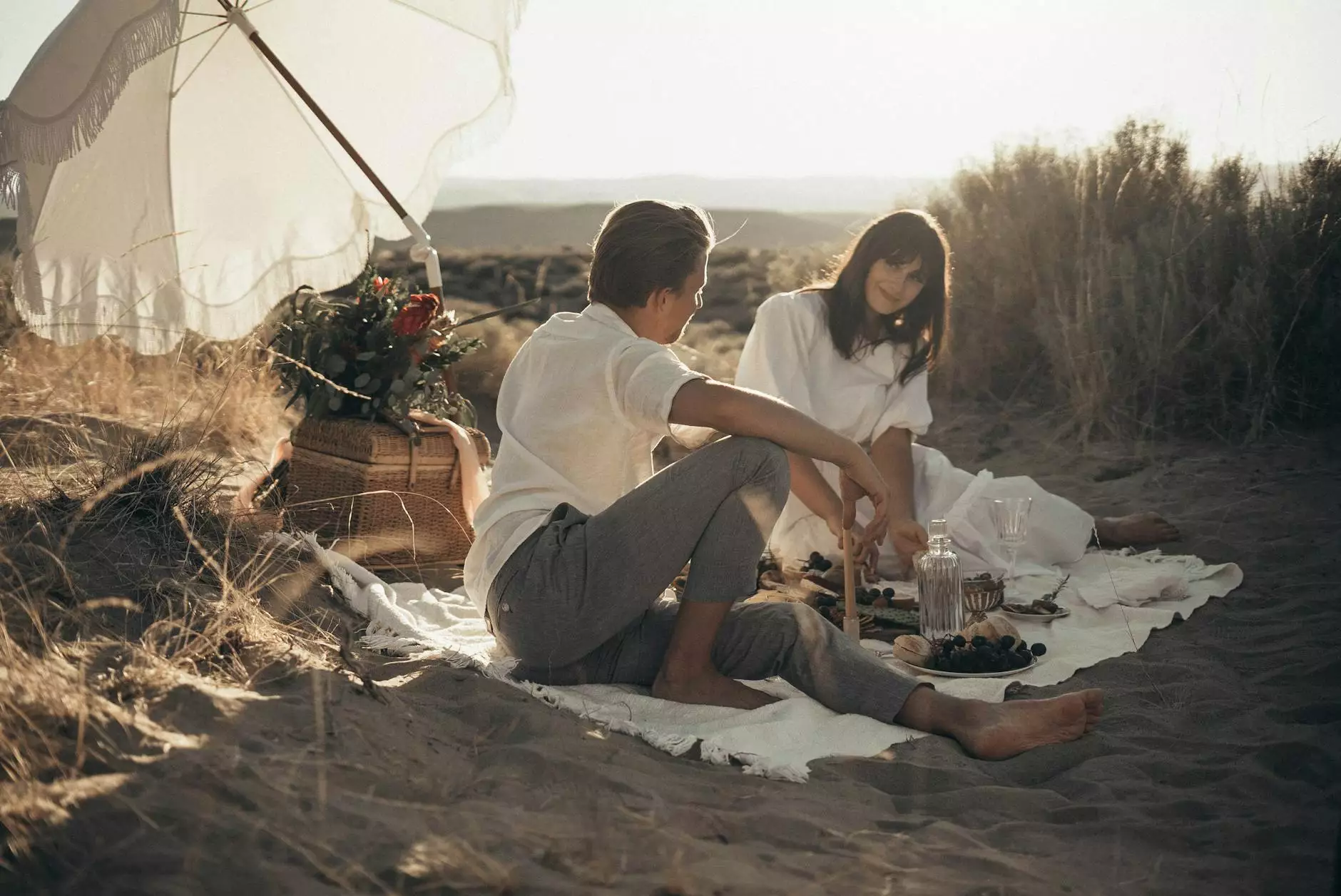
In today's fast-paced digital world, understanding how to convert milliseconds to date is a critical skill. Whether you are a web designer or a software developer, accurately handling time data is essential for building efficient applications. This article delves deeply into the methods and applications of converting milliseconds to date formats, ensuring you gain comprehensive insights.
What are Milliseconds?
Milliseconds are a unit of time that represents one thousandth of a second. In technical fields, particularly in programming and data analysis, time is frequently measured in milliseconds for precision and efficiency. For example, a complete second includes 1000 milliseconds:
- 1 second = 1000 milliseconds
- 1 minute = 60,000 milliseconds
- 1 hour = 3,600,000 milliseconds
The Importance of Time Conversion in Programming
In programming, data representing time is often stored as a timestamp in milliseconds. This is particularly true in languages such as JavaScript, Python, and Java. Since timestamps can be cumbersome, developers frequently need to convert milliseconds to date for better readability and functionality within applications.
Understanding how to handle these conversions effectively can streamline processes and enhance user experience, especially in web design and software development projects.
Methods to Convert Milliseconds to Date
There are multiple methods to convert milliseconds to date depending on the programming language you are using. Below are some prevalent approaches in various languages:
1. JavaScript
JavaScript makes it remarkably easy to convert milliseconds to date using the built-in Date object. Here’s how:
let milliseconds = 1609459200000; // Example timestamp let date = new Date(milliseconds); console.log(date.toString()); // Outputs: "Fri Jan 01 2021 00:00:00 GMT+0000 (Coordinated Universal Time)"2. Python
In Python, you can utilize the `datetime` module to make the conversion straightforward. Here’s a simple implementation:
import datetime milliseconds = 1609459200000 date = datetime.datetime.fromtimestamp(milliseconds / 1000.0) # Convert milliseconds to seconds print(date) # Outputs: 2021-01-01 00:00:003. Java
In Java, the Instant class from the Java 8 Time API provides a clean way to convert milliseconds to a date:
import java.util.Date; long milliseconds = 1609459200000L; Date date = new Date(milliseconds); System.out.println(date); // Outputs: Fri Jan 01 00:00:00 UTC 2021Applications of Milliseconds to Date Conversion
Converting milliseconds to date is not just a technical necessity; it has real-world applications across various industries:
- Web Applications: Many web applications display logs, events, and timelines that require human-readable date formats.
- Analytics: Data analytics platforms often operate on timestamps measured in milliseconds when tracking user activities or system performance.
- Database Management: Databases store and retrieve timestamped data that might need to be formatted for reports or user interfaces.
- APIs: When building APIs, returning time data in easy-to-read formats benefits client-side applications.
Best Practices for Handling Date Conversions
When working with date conversions, adhering to best practices ensures efficiency and accuracy:
1. Always Consider Time Zones
Time zone discrepancies can lead to confusion; therefore, always be mindful of the timezone settings in your applications. Utilize libraries (like Moment.js in JavaScript or pytz in Python) to ensure consistent time handling across regions.
2. Use Libraries for Complex Operations
Libraries can simplify the complexities associated with date and time manipulations. For instance, libraries like date-fns for JavaScript and pandas for Python offer a plethora of functions for handling dates and times effectively.
3. Validate Input Data
When converting timestamps, ensure that your input data is valid. This precaution prevents unnecessary errors and enhances the robustness of your code.
Conclusion
Being able to convert milliseconds to date is an invaluable skill for web designers and software developers. By understanding the methods, applications, and best practices for handling such conversions, you can improve your technical prowess and enhance your applications' functionality and user experiences. Whether it’s for displaying data effectively, analyzing performance, or integrating with APIs, mastering this skill is essential in today’s technology-centric landscape.
For more tips and tools on web design and software development, visit semalt.tools.